Hubspot Workflow is a very powerful tool that we can use to automate tasks. In this article, we will see how to update user properties using custom code in workflow.
Before you use custom code we need to configure the API key and fields (as per requirement) as shown below:
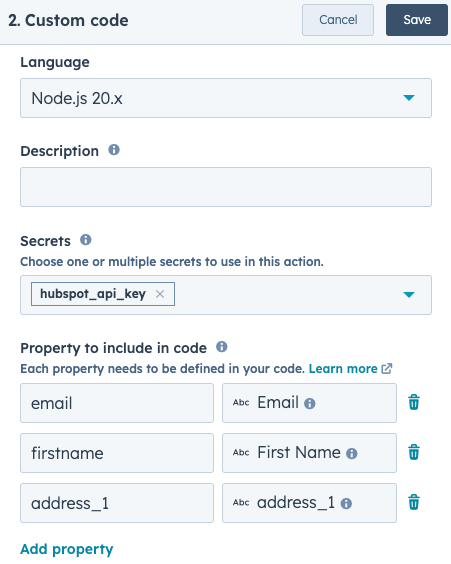
Now lets code:
const hubspot = require("@hubspot/api-client");
exports.main = async (event, callback) => {
const hubspotClient = new hubspot.Client({
accessToken: process.env.hubspot_api_key,
});
/*****
Use inputs to get data from any action in your workflow and use it in your code instead of having to use the HubSpot API.
*****/
const hs_object_id = event.inputFields["hs_object_id"];
const email = event.inputFields["email"];
const address_1 = event.inputFields["address_1"];
hubspotClient.crm.contacts.basicApi.update(hs_object_id, {
properties: {
address_1: address_1,
},
});
/*****
Use the callback function to output data that can be used in later actions in your workflow.
*****/
callback({
outputFields: {
email: email,
},
});
};